Interpolate alternatives and similar libraries
Based on the "Animation" category.
Alternatively, view Interpolate alternatives based on common mentions on social networks and blogs.
-
IBAnimatable
Design and prototype customized UI, interaction, navigation, transition and animation for App Store ready Apps in Interface Builder with IBAnimatable. -
SkeletonView
☠️ An elegant way to show users that something is happening and also prepare them to which contents they are awaiting -
AnimatedCollectionViewLayout
A UICollectionViewLayout subclass that adds custom transitions/animations to the UICollectionView without effecting your existing code. -
Presentation
:bookmark_tabs: Presentation helps you to make tutorials, release notes and animated pages. -
EasyAnimation
A Swift library to take the power of UIView.animateWithDuration(_:, animations:...) to a whole new level - layers, springs, chain-able animations and mixing view and layer animations together! -
Fluid Slider
:octocat:💧 A slider widget with a popup bubble displaying the precise value selected. Swift UI library made by @Ramotion -
ZoomTransitioning
ZoomTransitioning provides a custom transition with image zooming animation and swiping the screen edge. -
navigation-toolbar
:octocat: Navigation toolbar is a slide-modeled UI navigation controller made by @Ramotion -
CAROUSEL
List a collection of items in a horizontally scrolling view. A scaling factor controls the size of the items relative to the center. -
Garland View
:octocat: ≡ GarlandView seamlessly transitions between multiple lists of content. Swift UI library made by @Ramotion -
SPPerspective
Widgets iOS 14 animation with 3D and dynamic shadow. Customisable transform and duration. -
Numbers Animation
Numbers animation allows you to click on different numbers and accordingly it will animate numbers in a cool way. It has a very attractive UI and is very easy to use. -
CircularReveal
CircularReveal is a SwiftUI modifier that allows presenting views with a circular animation
WorkOS - The modern identity platform for B2B SaaS
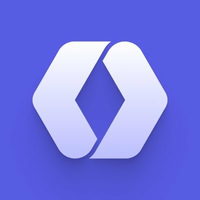
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of Interpolate or a related project?
README
Interpolate
Interpolate is a powerful Swift interpolation framework for creating interactive gesture-driven animations.
Usage
The :key: idea of Interpolate is - all animation is the interpolation of values over time.
To use Interpolate:
Import Interpolate at the top of your Swift file.
import Interpolate
Create an Interpolate object with a from value, a to value and an apply closure that applies the interpolation's result to the target object.
let colorChange = Interpolate(from: UIColor.white,
to: UIColor.red,
apply: { [weak self] (color) in
self?.view.backgroundColor = color
})
Alternatively, you can specify multiple values for the interpolation in an array. The Swift compiler might have issues to infer the type of the array so it's best to be explicit.
let colors: [UIColor] = [UIColor.white, UIColor.red, UIColor.green]
let colorChange = Interpolate(values: colors,
apply: { [weak self] (color) in
self?.view.backgroundColor = color
})
Next, you will need to define a way to translate your chosen gesture's progress to a percentage value (i.e. a CGFloat between 0.0 and 1.0).
For a gesture recognizer or delegate that reports every step of its progress (e.g. UIPanGestureRecognizer or a ScrollViewDidScroll) you can just apply the percentage directly to the Interpolate object:
@IBAction func handlePan(recognizer: UIPanGestureRecognizer) {
let translation = recognizer.translation(in: self.view)
let translatedCenterY = view.center.y + translation.y
let progress = translatedCenterY / self.view.bounds.size.height
colorChange.progress = progress
}
For other types of gesture recognizers that only report a beginning and an end (e.g. a UILongPressGestureRecognizer), you can animate directly to a target progress value with a given duration. For example:
@IBAction func handleLongPress(recognizer: UILongPressGestureRecognizer) {
switch recognizer.state {
case .began:
colorChange.animate(1.0, duration: 0.3)
case .cancelled, .ended, .failed:
colorChange.animate(0.0, duration: 0.3)
default: break
}
}
To stop an animation:
colorChange.stopAnimation()
When you are done with the interpolation altogether:
colorChange.invalidate()
Voila!
What can I interpolate?
Interpolate currently supports the interpolation of:
- CGPoint
- CGRect
- CGSize
- Double
- CGFloat
- Int
- NSNumber
- UIColor
- CGAffineTransform
- CATransform3D
- UIEdgeInsets
More types will be added over time.
Advanced usage
Interpolate is not just for dull linear interpolations.
For smoother animations, consider using any of the following functions: easeIn, easeOut, easeInOut and Spring.
// Spring interpolation
let shadowPosition = Interpolate(from: -shadowView.frame.size.width,
to: (self.view.bounds.size.width - shadowView.frame.size.width)/2,
function: SpringInterpolation(damping: 30.0, velocity: 0.0, mass: 1.0, stiffness: 100.0),
apply: { [weak self] (originX) in
self?.shadowView.frame.origin.x = originX
})
// Ease out interpolation
let groundPosition = Interpolate(from: CGPoint(x: 0, y: self.view.bounds.size.height),
to: CGPoint(x: 0, y: self.view.bounds.size.height - 150),
function: BasicInterpolation.easeOut,
apply: { [weak self] (origin) in
self?.groundView.frame.origin = origin
})
In fact, you can easily create and use your own interpolation function - all you need is an object that conforms to the InterpolationFunction protocol.
Setting up with CocoaPods
source 'https://github.com/CocoaPods/Specs.git'
pod 'Interpolate', '~> 1.3.0'
Setting up with Carthage
Carthage is a decentralized dependency manager that automates the process of adding frameworks to your Cocoa application.
You can install Carthage with Homebrew using the following command:
$ brew update
$ brew install carthage
To integrate Interpolate into your Xcode project using Carthage, specify it in your Cartfile
:
github "marmelroy/Interpolate"